Difference Between Encoding, Encrypting and Hashing
Similar with a few key differences.
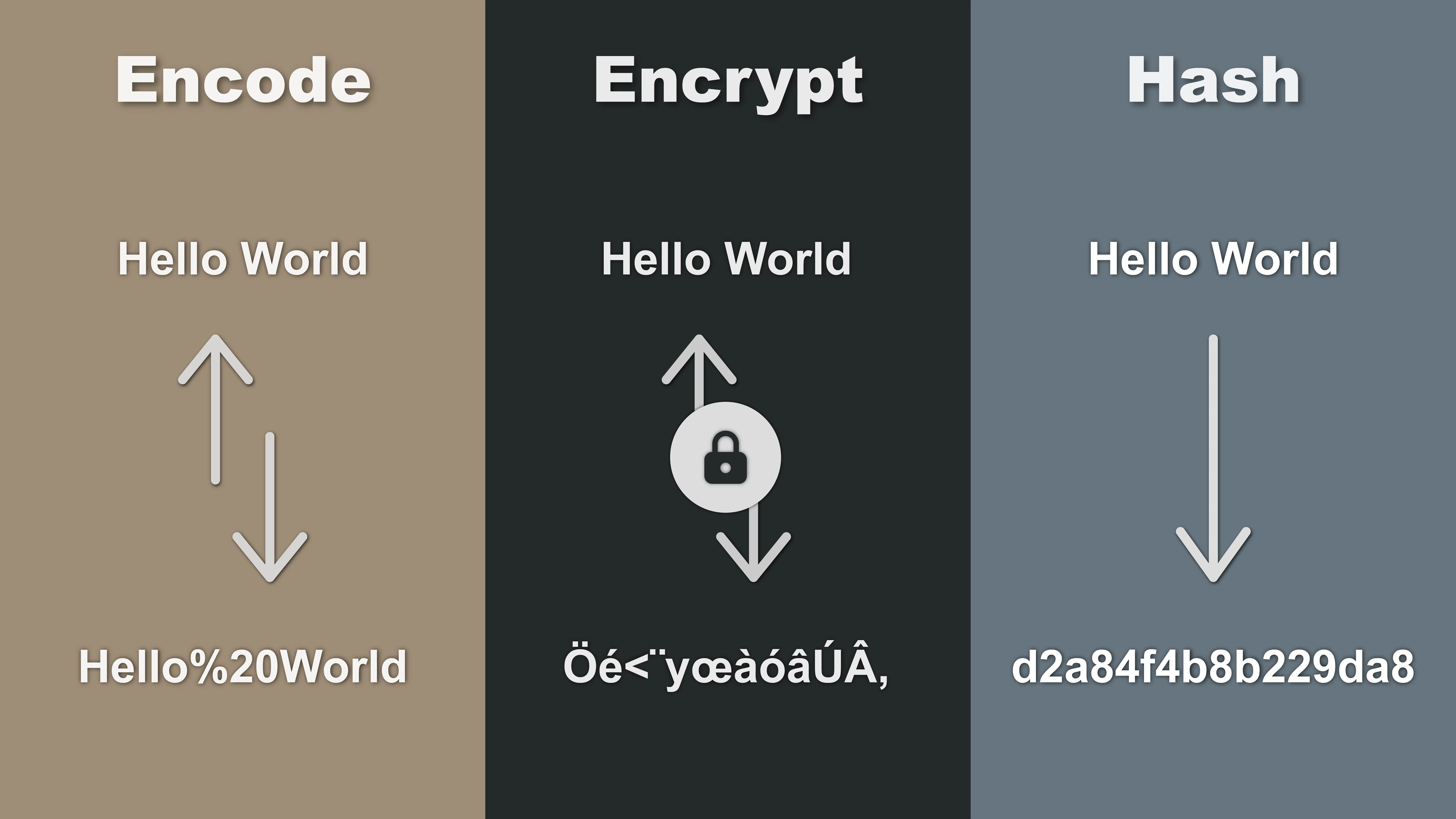
As a developer you’ve probably heard of encoding, encrypting, and hashing before.
I definitely have but I wasn’t completely aware of the all the differences so let’s talk about them.
Encoding
Encoding is simply converting data from one format to a different format to achieve compatibility. One of the most important details to remember is that encoding is designed to be reversible. If I encode some data to a format then it should be possible to decode it back to the original data.
Let’s take a look at a real world use case.
Try visiting the following URL in your browser: https://x.com/intent/post?text=hello
If you’re signed in then you should see Twitter open up a tweet draft pre-populated with the text hello
. This is because of the query param text
which is set to hello
. You can see an example screenshot below if you’re not signed in to Twitter.
Now what if we want to pre-populate with the text hello world
? Our intuition would say to change the query param to be ?text=hello world
but the issue here is that the space character between hello
and world
is not allowed in web URLs.
So whats the solution?
Browsers will use percent-encoding, also known as URL encoding, to make the text compatible with web URLs. This encoding is essentially replacing the incompatible character with a %
followed by the hexadecimal representation of the character’s ASCII value. So ?text=hello world
becomes ?text=hello%20world
and our tweet is then pre-populated correctly as seen below.
You can also see how this process is reversible too by just replacing the percent portions with their corresponding characters. You can see a table of the characters at MDN’s page about percent-encoding.
This is just one example of an encoding format and there are plenty more such as ASCII, UTF-8, UTF-16, UTF-32, and ISO-8859-1.
So to summarize just remember that encoding is meant to convert data from one format to another usually for compatibility and that the process is reversible.
Encryption
Encryption is the process of taking your data (also known as plaintext) and converting it to an unreadable version (also known as ciphertext) by using a cipher and a key.
A cipher is the algorithm used to produce the ciphertext and can be made public while the key is used by the cipher to encrypt the data and must remain private.
The main difference between encryption and encoding is that encryption makes the output unreadable and is reversible only if you have the key.
Let’s take a look at an example of encryption.
Here are some commands I ran in my terminal to encrypt a simple text file with the OpenSSL library.
# Write "hello world" to a text file called hello.txt
echo "hello world" > hello.txt
# This is where the encryption happens, lets explain the command
# openssl enc -> Use the encryption function of the openssl library
# -aes-256-cbc -> Specify the cipher to use (AES with 256-bit key in CBC mode)
# -pbkdf2 -> Password Based Key Derivation Function 2, specify which function to use to generate the key
# -salt -> Use a random "salt", which is some random characters, in the pbkdf2 to generate a unique key each time
# -in -> File whose content you want to encrypt
# -out -> File to output the encrypted content to
# -pass pass:supersecret -> specifies the password to use for key derivation (must start with "pass:" followed by the actual password)
openssl enc -aes-256-cbc -salt -pbkdf2 -in hello.txt -out encrypted_hello.txt -pass pass:supersecret
# Print out the content of the encrypted file
cat encrypted_hello.txt
# Encrypted Output: Salted__ƒ˚∏ª⁄+¨Æflfl∆ºnÑ ÷˚∞≥J2É
As you’ve noticed the encrypted output is gibberish to us. You can also add the -base64
option to the command so that the output is encoded to Base64 if you need it.
So it would look like the following:
# Notice we've added the -base64 option
openssl enc -aes-256-cbc -salt -pbkdf2 -base64 -in hello.txt -out encrypted_hello.txt -pass pass:supersecret
cat encrypted_hello.txt
# Encrypted Output in Base64: U2FsdGVkX1+ngxMkZzGeZMZvtVm9tg8/vhKe9TGNylU=
Now here is the command to decrypt the file.
# Notice we add the "-d" flag for decryption
# The other change is that the input is now the encrypted file and the output is a new file that will contain the plaintext
openssl enc -d -aes-256-cbc -salt -pbkdf2 -in encrypted_hello.txt -out decrypted_hello.txt -pass pass:supersecret
cat decrypted_hello.txt
# Decrypted Output: hello world
Now we’ve successfully encrypted and decrypted a file!
Just a couple small notes about the -salt
flag. This flag is enabled by default but I added -salt
just to be explicit. Using a salt is optional and you can omit the salt by adding the -nosalt
flag but this is not recommended as it is less secure. The salt adds some randomness to the derived key so that if you encrypt the same file with the same password you get a different ciphertext each time since the salt changes.
Also worth noting that we went through a simple example of symmetric encryption (encryption with one private key) but there is also asymmetric encryption (encryption with public and private key) where the public key is made available to anyone to use for encrypting data but then only the private key, which is still a secret, can be used to decrypt that data.
So to summarize just remember that encryption is meant to convert plaintext to ciphertext for security purposes and that the process is reversible as long as you know the used cipher and have the private key.
Hashing
Hashing takes in an input and returns a unique fixed-size string of characters known as the hash value or digest.
The most important difference compared to encoding and encryption is that hashing is not reversible. So if you have a hash value there is no way to get the original data back from that value.
Let’s take a look at the hashing example below.
# Write the string "Hello" to a file
echo "Hello" > sample.txt
# Use the SHA-256 hashing algorithm on the file we just created
shasum -a 256 sample.txt
# Output: 66a045b452102c59d840ec097d59d9467e13a3f34f6494e539ffd32c1bb35f18
# Write the string "Hello ", which has an extra space character at the end, to a new file
echo "Hello " > sample2.txt
# Use the SHA-256 hashing algorithm on the new file
shasum -a 256 sample2.txt
# Output: a4cfc596d52c95b51ab28b4def04f83da35cd3a4c6db1b6001d4d0fa41809221
Notice how even though the content was different by just one space character the hash value was completely different. This makes hashing great for detecting file corruption since if the file is modified even slightly then it will result in a completely different hash.
Hashing is also great for storing passwords for any applications you may build. When a user signs up you can save the hashed version of their password in your database so next time they login you hash the password they entered and compare it to the saved hash in your database. This way you never have to store the original password value.
I also want to briefly touch on rainbow tables which are tables that map known hashes to their original value. So if you have a hash you can check to see if it exists in a rainbow table and get the value of it. You can visit CrackStation and Hashes.com, which are two well known rainbow tables, to try it out. To be clear rainbow tables are not reversing the hashing process but instead just hashing a bunch of different values and saving their hash into a table.
So to summarize just remember that hashing returns a unique fixed-size string of characters known as the hash value or digest and that the process is not reversible.
Summary
Here’s a quick summary of each of the methods we learned today.
Encoding
- Converts data from one format to another usually for compatibility.
- Reversible and is not designed to secure data.
Encryption
- Converts plaintext to ciphertext using a cipher and a key for security.
- Reversible if you know the cipher and the private key.
Hashing
- Outputs a unique hash value and is great for checking that data has not been corrupted.
- Not reversible and any slight changes in data will produce a completely different hash value.
That’s all I have to share for now.
Feel free to @ me on twitter if you found this post helpful or if you have something you’d like to see me write about.
Until next time, keep improving and never stop learning.
— Mo